I use the Markdown-it engine to implement the feature set I find useful to have for my articles:
- Easy to use figures with indexing and referencing.
- Code snippets with optional titles and the ability to copy the cope with one button.
- External link handling.
- Section dividers with optional titles.
- Message boxes.
- Anchor links.
I used the following software components:
Package | Version | Description |
---|---|---|
markdown-it | 8.3.1 | Core engine. |
markdown-it-anchor | 4.0.0 | Anchor links for header. |
markdown-it-container | 2.0.0 | Custom containers with predefined classes. |
markdown-it-custom-block | 0.1.0 | Custom blocks with custom renderers. |
markdown-it-external-links | 0.0.6 | External link handling. |
markdown-it-footnote | 3.0.1 | Footnote system. |
Since I am using Metalsmith for my blog, I was implemented the custom Markdown system as a Metalsmith plugin with a few helper function. The sources for the whole system can be found on my site’s GitHub repository.
Figure system
My figure system uses the markdown-it-custom-block plugin to define the necessary custom renderer function as follows:
const md = new MarkdownIt()
md.use(mdCustomBlock, {
img (raw) {
const [index, alt, width, url] = raw.split('#')
return `<figure id="fig${index}">
<img width=${width} src="/assets/images/${url}" alt="${alt}">
<figcaption>Fig ${index}: ${alt}</figcaption>
</figure>`
}
})
That code snippet will define a custom @[img](index#title#width#image_url)
block that can be used to insert figures. This one-liner will result the following html code:
<figure id="fig${index}">
<img width=${width} src="/assets/images/${image_url}" alt="${title}">
<figcaption>Fig ${index}: ${title}</figcaption>
</figure>
Using a bit of CSS wizardry will create automatic wrapper-less borders around the image blocks:
figure {
position: relative;
border: none;
border-bottom: 1px solid palette(Black, Dividers);
width: var(--content-width);
left: - $content-padding;
margin: 0;
display: flex;
flex-direction: column;
align-items: center;
img {
max-width: 740px;
margin: 20px 0px 8px;
}
figcaption {
font-size: 0.9em;
font-style: italic;
margin-bottom: 10px;
}
}
:not(figure)+figure {
border-top: 1px solid palette(Black, Dividers);
}
Figures example
The following markup will be rendered as a figure group containing three images. Each images will have its own figure id, title, and a hidden anchor /#fig<number>
link for later references.
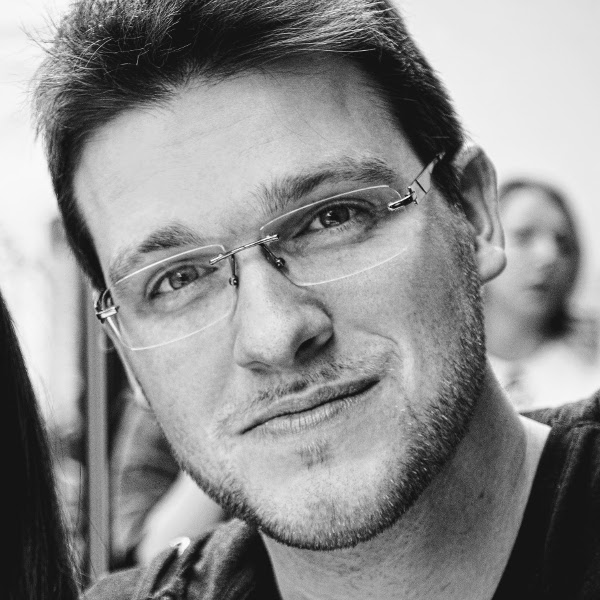
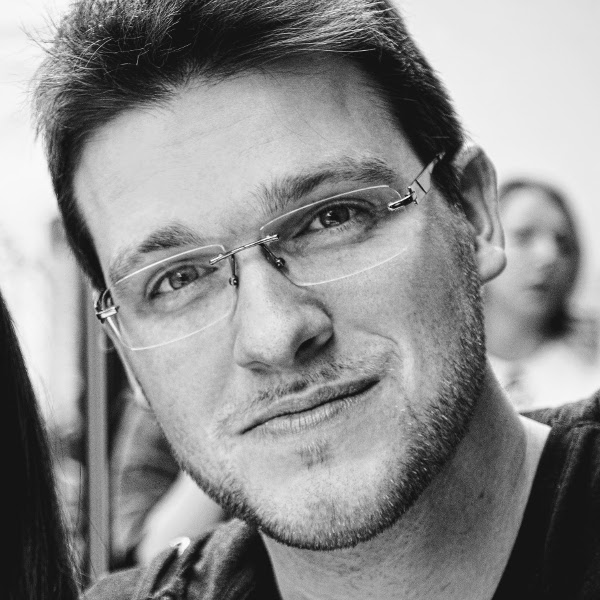
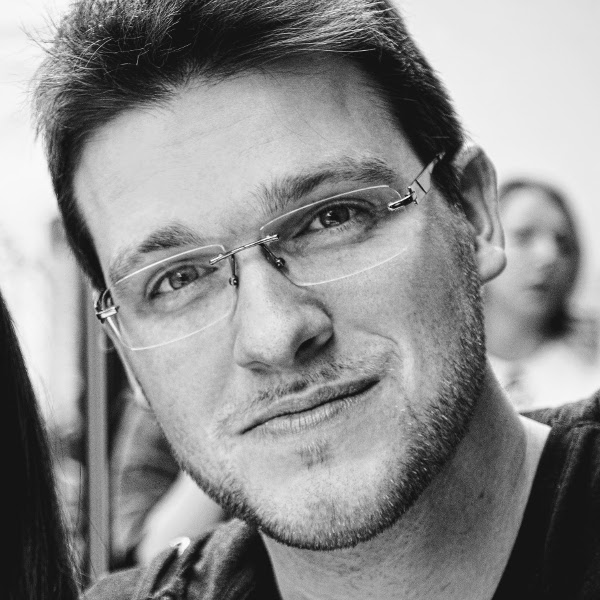
Figure syntax@[img](1#Test figure 1.#160#tibor2017-600.jpg) @[img](2#Test figure 2.#160#tibor2017-600.jpg) @[img](3#Test figure 3.#160#tibor2017-600.jpg)
Divider
Similarly to the figures, the dividers also implemented with the markdown-it-custom-block plugin.
const md = new MarkdownIt()
md.use(mdCustomBlock, {
divider (text) {
return `<div class="divider">${text}</div>`
}
})
Divider example
Divider syntax@[divider](My first divider) @[divider](My second divider)
Message boxes
I like to use message boxes to highlight sime important sections.
This is a success message.
This is a info message.
This is a warning message.
This is a danger message.
Message box syntax::: success This is a success message. ::: ::: info This is a info message. ::: ::: warning This is a warning message. ::: ::: danger This is a danger message. :::